Python is
the language of the Python Interpreter and those who can converse with it. An
individual who can speak Python is known as a Pythonista. It is a very uncommon
skill, and nearly all known Pythonistas use software initially developed by
Guido van Rossum.
When we are at the python interpreter, it displays chevron
prompt (>>>) on the terminal, where it is asking you “What’s next??”.
How do we speak the language of python??
This must be the question on most of your minds when trying
to learn the language initially. Just like the English Language, we will first
start of by learning words, sentences and then paragraphs in python.
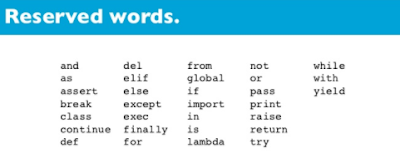
Constants and variables are other literals that are
supported by python. “Constants”, as the word suggests they’re fixed values such as numbers, letters,
and strings, where their value does not change throughout the scope of
the program. A variable is a
named place in the memory where a programmer can store data and later retrieve
the data using the variable “name”.
Since we programmers are given a choice in how we choose our
variable names, there is a bit of “best practice”, we name variables to help us
remember what we intend to store in them (“mnemonic” = “memory aid”). This can
confuse beginning students because well-named variables often “sound” so good
that they must be keywords. There are rules that have to be followed while
naming a variable so as to reduce ambiguity for the interpreter, and they’re:
- Must start with a letter or underscore _
- Must consist of letters, numbers, and underscores
- Case Sensitive
- Cannot be Reserved Word.
Next comes sentences, or here we are specifically talking
about how do we code instruction in python. For simpler understanding let’s
start off with arithmetic expressions.
Assignment
Statement: x=2
Arithmetic
assignment: x=x+2
Output
Statement: print(x)
So, what all did we come across in the previous three
instructions, operators, constants, variables and function.
Numeric Expressions that are used in python are mentioned
below, //pic of operator operation table
When we have so many operators for numeric expressions to
perform simple calculation, there must be a way python must know which of them
to do first, so to address this situation we will have to learn about the
concept of operator precedence, i.e. which operator takes precedence over the
others.
Highest
precedence rule to lowest precedence rule:
- Parentheses are always respected
- Exponentiation (raise to a power)
- Multiplication, Division, and Remainder
- Addition and Subtraction
- Left to right
Note: Remember in python3 when you perform integer division
it produces a floating-point result.
Now lastly let’s see how to write paragraphs, Interactive
python is good for experimenting programs of 3 to 4 lines, but most programs
are much longer than that, so we type our python scripts in a file using an
editor and then we tell python to run those commands that we have coded in the
file. As a convention we add .py extension for python files.
Sequential flow of execution is followed by python
interpreter unless it comes across some branching, looping or conditional
statement. This means to say that when a program is running, that the flow of
execution occurs from one step to the next. As programmers we set paths for the
program to follow.

There are certain scenario when the programmer would
explicitly like to typecast a named space and to do so there are some built-in
functions available to us, such as:
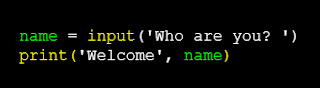
Let’s say we want to read a number from the user then we
must convert the string object to integer or float using the typecasting
function which we have seen earlier.
Comments in python are prefixed by the ‘#’ symbol, when the
interpreter comes across a line prefixed with ‘#’ then it doesn’t execute it or
ignores it as comment. As a beginner in programming you might think why do we
need to write comments in a program, the reasons are,
- Describe
what is going to happen in a sequence of code
-
Document who wrote the code or other ancillary information
- Turn
off a line of code - perhaps temporarily
So now we have come to the end of introduction in python,
and at this checkpoint we have pictured the edges of a big canvas about python
and we will focus on the details of this canvas in the next post. Stay tuned
for the next post on the topic of conditional statements and iterations, until
then guys enjoy your time browsing through other posts in the blog!!
Post a Comment
Click to see the code!
To insert emoticon you must added at least one space before the code.